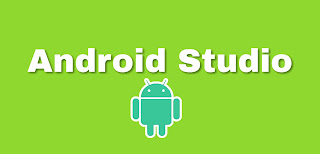
Handle Clicks on Button in Android Studio
There are two methods to handle clicks on button in android studio. In the first we create a onClick method and add it to the button XML code. Then we create that method with the name provided in XML code (android:onClick="method_name"). Now put your code you want to run after the click inside this method. Second we create a button and provide a id to it. Then we call method findViewById(R.id.given_id). This method will return the object with this id.In this case it is a Button. Call setOnClickListner() method on the button object. This method takes one argument View.OnClickListner class object with overridden method onClick(). Put your code inside this method to be run on click.
Main Activity:
XML Code -
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
>
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
android:gravity="center"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent">
<Button
android:onClick="buttonClick"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="onClick"
android:textAllCaps="false"
android:textSize="40dp"
android:padding="10dp"
android:textColor="#ffffff"
android:background="@color/blue"/>
<Button
android:id="@+id/myButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="SetOnClickListner"
android:textAllCaps="false"
android:textSize="40dp"
android:layout_marginTop="20dp"
android:padding="10dp"
android:textColor="#ffffff"
android:background="@color/blue"/>
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
JAVA Code -
package com.bharat_putra_tech.testapp;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.os.Handler;
import android.view.View;
import android.widget.Button;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button mybutton = findViewById(R.id.myButton);
mybutton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent i = new Intent(getApplicationContext() , Main2Activity.class);
startActivity(i);
}
});
}
public void buttonClick(View view) {
Intent i = new Intent(this, Main2Activity.class);
startActivity(i);
}
}
Main Activity 2:
XML Code -
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".Main2Activity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Wellcome"
android:textColor="@color/blue"
android:textSize="40dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toBottomOf="parent"/>
</androidx.constraintlayout.widget.ConstraintLayout>
Result -
YouTube video link
No comments:
Post a Comment