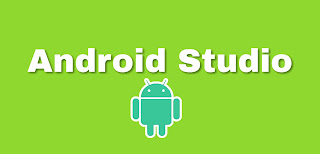
Android Animation : Zoom in and Zoom out Animation
This is third tutorial of android animation. In this tutorial we will learn about android view animation. We will create zoom in and zoom out animation using xml.
Anim Directory:
First of all create anim resource files as zoom_in.xml and zoom_out.xml.(If you do not have anim resource than create it first).
If you don't know how to create a anim resource directory than watch this tutorial.
Codes:
1. zoom_in.xml
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android">
<scale
android:duration="1200"
android:pivotY="50%"
android:pivotX="50%"
android:fromYScale="1"
android:toYScale="3"
android:fromXScale="1"
android:toXScale="3"/>
</set>
2. zoom_out.xml
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android">
<scale
android:duration="1200"
android:pivotY="50%"
android:pivotX="50%"
android:fromYScale="1"
android:toYScale="0.3"
android:fromXScale="1"
android:toXScale="0.3"/>
</set>
3. activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:gravity="center"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toBottomOf="parent">
<ImageView
android:id="@+id/logo1"
android:layout_width="200dp"
android:layout_height="100dp"
android:src="@drawable/logo"
android:layout_marginBottom="60dp"/>
<Button
android:id="@+id/zoomin"
android:layout_width="200dp"
android:layout_height="70dp"
android:text="Zoom IN"
android:textSize="30dp"
android:background="@drawable/capsule"
android:layout_marginBottom="20dp"/>
<Button
android:id="@+id/zoomout"
android:layout_width="200dp"
android:layout_height="70dp"
android:text="Zoom OUT"
android:textSize="30dp"
android:background="@drawable/capsule"
android:layout_marginBottom="20dp"/>
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
3. ActivityMain.java
package com.bharat_putra_tech.testapp;
import androidx.appcompat.app.AppCompatActivity;
import android.animation.AnimatorListenerAdapter;
import android.os.Bundle;
import android.view.View;
import android.view.animation.Animation;
import android.view.animation.AnimationUtils;
import android.widget.Button;
import android.widget.ImageView;
public class MainActivity extends AppCompatActivity {
Animation zoom_in,zoom_out;
ImageView logo;
Button in,out;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
logo = findViewById(R.id.logo1);
in = findViewById(R.id.zoomin);
out = findViewById(R.id.zoomout);
in.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
zoom_in = AnimationUtils.loadAnimation(getApplicationContext(),R.anim.zoom_in);
logo.setVisibility(View.VISIBLE);
logo.startAnimation(zoom_in);
}
});
out.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
zoom_out = AnimationUtils.loadAnimation(getApplicationContext(),R.anim.zoom_out);
logo.setVisibility(View.VISIBLE);
logo.startAnimation(zoom_out);
}
});
}
}
Result :
Video Tutorial:
Great setup for learning programs 🔥
ReplyDeleteTHANKS
Delete