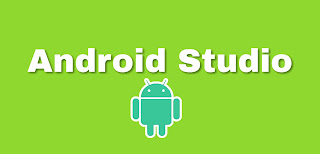
Splash Screen Android Studio
Splash Screen is a welcome screen. It is used in all kind of apps and games. We will create a splash screen using Handler class. There is a method in handler class postDelayed(). It takes two arguments fist one is a Runnable Object and second is time in milli-seconds. This method runs the code in run method inside Runnable after given time.
Main Activity:
XML Code -
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
android:background="@drawable/splash"
>
<LinearLayout
android:layout_width="400dp"
android:layout_height="300dp"
android:orientation="vertical"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintBottom_toBottomOf="parent">
<ImageView
android:layout_width="400dp"
android:layout_height="200dp"
android:src="@drawable/logo"/>
<ImageView
android:layout_width="400dp"
android:layout_height="100dp"
android:src="@drawable/name"/>
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
JAVA Code -
package com.bharat_putra_tech.testapp;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.os.Handler;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Handler h = new Handler();
h.postDelayed(new Runnable() {
@Override
public void run() {
Intent i = new Intent(getApplicationContext(), Main2Activity.class);
startActivity(i);
}
},3000);
}
}
Main Activity 2:
XML Code -
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".Main2Activity">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Wellcome\nyou have successfully created splash screen"
android:textColor="@color/green"
android:textSize="30dp"
android:textAlignment="center"
android:gravity="center"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toBottomOf="parent"/>
</androidx.constraintlayout.widget.ConstraintLayout>
Result -
YouTube video link
No comments:
Post a Comment