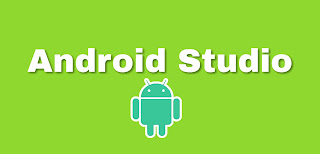
Change Attributes of TextView Dynamically in Android Studio
In this article we will learn how we can change attributes of TextView on runtime. Suppose I am creating a app in which I want to show that anything which not expected in red color text. Or I want to change text size on runtime or I want to change the text of the View. Then it is usefull.
Android provides methods which can be used to set different attributes of the view. We will use method setText() to change the text of the view. We can pass the text to be set to the view as a String. setTextColor() helps us to set color of the text. It takes one argument which is a no related to a color. We have used Color.RED to assign the color. There are many colors available in this Color class. Now we use setTextSize() method to set the size on runtime. It takes one float argument as size.
Main Activity:
XML Code -
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
>
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
android:gravity="center"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toBottomOf="parent">
<TextView
android:id="@+id/myText"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello"
android:textSize="40dp"
android:textColor="@color/blue"/>
<Button
android:id="@+id/text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Text"
android:textAllCaps="false"
android:textSize="30dp"
android:background="@color/green"
android:layout_marginTop="20dp"/>
<Button
android:id="@+id/color"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Color"
android:textAllCaps="false"
android:textSize="30dp"
android:background="@color/green"
android:layout_marginTop="20dp"/>
<Button
android:id="@+id/size"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Size"
android:textAllCaps="false"
android:textSize="30dp"
android:background="@color/green"
android:layout_marginTop="20dp"/>
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
JAVA Code -
package com.bharat_putra_tech.testapp;
import androidx.appcompat.app.AppCompatActivity;
import android.graphics.Color;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
final TextView mytext = findViewById(R.id.myText);
Button text = findViewById(R.id.text);
Button color = findViewById(R.id.color);
Button size = findViewById(R.id.size);
text.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
mytext.setText("Wellcome");
}
});
color.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
mytext.setTextColor(Color.RED);
}
});
size.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
mytext.setTextSize(mytext.getTextSize()+1);
}
});
}
}
Result -
YouTube video link
👍👍👍
ReplyDeleteThanks for your support
Delete