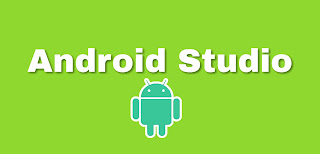
Disable Button when Edit Text is empty, TextWatcher - Android Studio
In this article we will learn how to create a button which will be disabled if the edit text field are empty. We will use TextWatcher interface. We will create a instance of this interface and override the methods. We will write code in onTextChange() method. This method will be called when text is changed. We will check that both fields are not empty, then set button enabled. We will use setEnabled(true) on button object to make it enabled. Else we will use same method with attribute false in order to disable the button. Following is the full code
Main Activity:
XML Code -
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="10dp"
android:gravity="center"
tools:context=".Main2Activity">
<EditText
android:id="@+id/name"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@drawable/background2"
android:textSize="30dp"
android:padding="10dp"
android:hint="Name"
android:textColor="@color/blue"/>
<EditText
android:id="@+id/age"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@drawable/background2"
android:textSize="30dp"
android:padding="10dp"
android:hint="Age"
android:layout_marginTop="20dp"
android:textColor="@color/blue"/>
<Button
android:id="@+id/submit_btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Submit"
android:layout_marginTop="20dp"
android:textAllCaps="false"
android:textSize="30dp"
android:padding="10dp"/>
</LinearLayout>
JAVA Code -
package com.example.test;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.text.Editable;
import android.text.TextWatcher;
import android.widget.Button;
import android.widget.EditText;
public class Main2Activity extends AppCompatActivity {
EditText name, age;
Button submit;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main2);
name = findViewById(R.id.name);
age = findViewById(R.id.age);
submit = findViewById(R.id.submit_btn);
submit.setEnabled(false);
name.addTextChangedListener(textWatcher);
age.addTextChangedListener(textWatcher);
}
private TextWatcher textWatcher = new TextWatcher() {
@Override
public void beforeTextChanged(CharSequence s, int start, int count, int after) {
}
@Override
public void onTextChanged(CharSequence s, int start, int before, int count) {
String n = name.getText().toString();
String a = age.getText().toString();
if(!n.isEmpty() && !a.isEmpty()){
//both are not empty
submit.setEnabled(true);
}else{
submit.setEnabled(false);
}
}
@Override
public void afterTextChanged(Editable s) {
}
};
}
Result -
YouTube video link
No comments:
Post a Comment