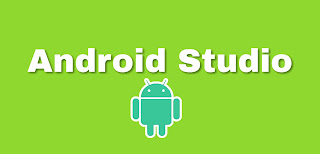
Customize Design of Button in Android Studio
A beautiful UI is essential for any app or game. In this article we have provided a code for some beautiful button design. This is done by creating xml files. We create drawable resource file in drawable folder. This design is used for providing background to any kind of View not only buttons. We can create beautiful buttons using graphics also, but graphics takes a large file size and in the result your app size will unnessasery increase. So we use this xml resource files insted of graphics.
Drawable resource files Codes:
stroke.xml -
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android">
<solid
android:color="#ffffff"/>
<stroke
android:width="4dp"
android:color="@color/blue"/>
</shape>
capsule.xml -
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android">
<size
android:height="50dp"
android:width="100dp"/>
<stroke
android:width="3dp"
android:color="@color/blue"/>
<solid
android:color="#ffffff"/>
<corners
android:radius="50dp"/>
</shape>
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android">
<gradient
android:startColor="@color/skyblue"
android:endColor="@color/blue"/>
<size
android:height="50dp"
android:width="100dp"/>
<corners
android:radius="10dp"/>
</shape>
<?xml version="1.0" encoding="utf-8"?>
<shape android:shape="oval" xmlns:android="http://schemas.android.com/apk/res/android">
<size
android:height="50dp"
android:width="100dp"/>
<solid
android:color="@color/blue"/>
</shape>
<?xml version="1.0" encoding="utf-8"?>
<shape
xmlns:android="http://schemas.android.com/apk/res/android">
<solid
android:color="@color/blue"/>
<size
android:height="100dp"
android:width="100dp"/>
<corners
android:radius="50dp"/>
</shape>
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android">
<size
android:height="50dp"
android:width="100dp"/>
<gradient
android:startColor="@color/skyblue"
android:endColor="@color/blue"/>
<corners
android:topLeftRadius="20dp"
android:bottomLeftRadius="5dp"
android:bottomRightRadius="20dp"
android:topRightRadius="5dp"/>
</shape>
XML Code for UI:
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:gravity="center"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toBottomOf="parent">
<Button
android:layout_width="100dp"
android:layout_height="50dp"
android:text="stroke"
android:background="@drawable/stroke"/>
<Button
android:layout_marginTop="10dp"
android:layout_width="100dp"
android:layout_height="50dp"
android:text="capsule"
android:background="@drawable/capsule"/>
<Button
android:layout_marginTop="10dp"
android:layout_width="100dp"
android:layout_height="50dp"
android:text="rounded"
android:textColor="#ffffff"
android:background="@drawable/rounded_corner"/>
<Button
android:layout_marginTop="10dp"
android:layout_width="100dp"
android:layout_height="50dp"
android:text="oval"
android:textColor="#ffffff"
android:background="@drawable/oval"/>
<Button
android:layout_marginTop="10dp"
android:layout_width="100dp"
android:layout_height="50dp"
android:text="mys tyle"
android:textColor="#ffffff"
android:background="@drawable/mystyle"/>
<Button
android:layout_marginTop="10dp"
android:layout_width="100dp"
android:layout_height="100dp"
android:text="circle"
android:textColor="#ffffff"
android:background="@drawable/circle"/>
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
Result -
YouTube video link
No comments:
Post a Comment