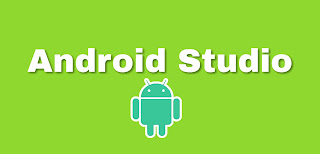
Share pre-formatted text message from App - Android Studio
In this article we will learn how to share a pre-formatted text message from app on social media platforms, like whatsapp, instagram, gmail etc. This method is not working in case of facebook.
We will use intent for this task. First, we set Action of the intent through constructor like this Intent(Intent.ACTION_SEND). To share a message or file we have to set action to this Intent.ACTION_SEND. Now set type, for sharing a text message we use type "text/plain". Now put text using method putExtra(). This method take two argument, first is name (String) and second is Data. We have to use constants for name, for text message we use name Intent.EXTRA_TEXT. Pass the message you want to share as second argument. Now call startActivity() method and pass this intent.
Example Code:
XML Code -
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="10dp"
android:gravity="center"
tools:context=".Main2Activity"
android:background="@color/blue">
<Button
android:id="@+id/share_btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Share"
android:textSize="40dp"
android:paddingLeft="10dp"
android:paddingRight="10dp"
android:background="@drawable/corner"/>
</LinearLayout>
JAVA Code -
package com.example.test;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
public class Main2Activity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main2);
Button sharebutton = findViewById(R.id.share_btn);
sharebutton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(Intent.ACTION_SEND);
intent.setType("text/plain");
intent.putExtra(Intent.EXTRA_TEXT,"This is the pre-formatted message to send.");
startActivity(intent);
}
});
}
}
Result -
YouTube video link
No comments:
Post a Comment