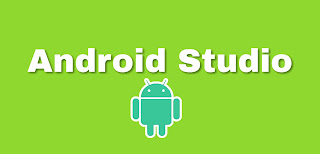
Android Animation : Move Animation
This is fourth tutorial of android animation. In this tutorial we will learn about android view animation. We will create move up down left and right animation using xml.
Anim Directory:
First of all create anim resource files as move_up.xml , move_down.xml , move_left.xml and move_right.xml. (If you do not have anim resource than create it first).
If you don't know how to create a anim resource directory than watch this tutorial.
Codes:
1. move_up.xml
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android">
<translate
android:duration="2000"
android:fromYDelta="100%p"
android:toYDelta="0%p"/>
</set>
2. move_down.xml
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android">
<translate
android:duration="2000"
android:fromYDelta="0%p"
android:toYDelta="100%p"/>
</set>
3. move_left.xml
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android">
<translate
android:duration="2000"
android:fromXDelta="100%p"
android:toXDelta="0%p"/>
</set>
4. move_right.xml
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android">
<translate
android:duration="2000"
android:fromXDelta="0%p"
android:toXDelta="100%p"/>
</set>
5. activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:gravity="center"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toBottomOf="parent">
<ImageView
android:id="@+id/logo1"
android:layout_width="200dp"
android:layout_height="100dp"
android:src="@drawable/logo"
android:layout_marginBottom="60dp"/>
<Button
android:id="@+id/up"
android:layout_width="200dp"
android:layout_height="50dp"
android:text="up"
android:textSize="30dp"
android:background="@drawable/capsule"
android:layout_marginBottom="20dp"/>
<Button
android:id="@+id/down"
android:layout_width="200dp"
android:layout_height="50dp"
android:text="down"
android:textSize="30dp"
android:background="@drawable/capsule"
android:layout_marginBottom="20dp"/>
<Button
android:id="@+id/left"
android:layout_width="200dp"
android:layout_height="50dp"
android:text="left"
android:textSize="30dp"
android:background="@drawable/capsule"
android:layout_marginBottom="20dp"/>
<Button
android:id="@+id/right"
android:layout_width="200dp"
android:layout_height="50dp"
android:text="right"
android:textSize="30dp"
android:background="@drawable/capsule"
android:layout_marginBottom="20dp"/>
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
6. ActivityMain.java
package com.bharat_putra_tech.testapp;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.view.animation.Animation;
import android.view.animation.AnimationUtils;
import android.widget.Button;
import android.widget.ImageView;
public class MainActivity extends AppCompatActivity {
Animation move_up,move_down,move_left,move_right;
Button up,down,left,right;
ImageView logo;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
logo = findViewById(R.id.logo1);
up = findViewById(R.id.up);
down = findViewById(R.id.down);
left = findViewById(R.id.left);
right = findViewById(R.id.right);
up.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
move_up = AnimationUtils.loadAnimation(getApplicationContext(),R.anim.move_up);
logo.setVisibility(View.VISIBLE);
logo.startAnimation(move_up);
}
});
down.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
move_down = AnimationUtils.loadAnimation(getApplicationContext(),R.anim.move_down);
logo.setVisibility(View.VISIBLE);
logo.startAnimation(move_down);
}
});
left.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
move_left = AnimationUtils.loadAnimation(getApplicationContext(),R.anim.move_left);
logo.setVisibility(View.VISIBLE);
logo.startAnimation(move_left);
}
});
right.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
move_right = AnimationUtils.loadAnimation(getApplicationContext(),R.anim.move_right);
logo.setVisibility(View.VISIBLE);
logo.startAnimation(move_right);
}
});
}
}
Result :
Video Tutorial:
No comments:
Post a Comment